Suppose you have a series of Linux commands, which you need to repeat multiple times. You can either write the commands 1,000 times and curse your fate, or you can write a small and simple bash script in Linux to automate this tedious task. In this article, we will discuss what is a bash script, its advantages, and how to write a bash script in Linux.
What is Bash Script in Linux
Just like a movie script gives actors an idea of how to act out a particular scene. In the same way, a Bash script is a file containing a series of Linux commands which when executed, work like they have been typed in the shell prompt, thereby, automating your workflow. All bash scripts have a .sh extension, a “shebang line” – #/bin/bash
, and can be directly run via the shell prompt.
In short, a bash script groups all the commands you need to execute individually for a particular task. Now that you know what is a bash script in Linux, let us now see its advantages.
Advantages of Using Bash Scripts in Linux
Bash script in general is a powerful and versatile tool making it an integral part of every system administrator, developer, and power user’s arsenal. Here are some key advantages of using bash scripts:
- Automation: This is possibly the most sought-after advantage of using bash scripts. With bash scripts, you get the power to automate even the most boring, repetitive tasks, thereby reducing effort, saving time, and minimizing errors.
- Flexibility: You can create custom bash scripts to suit your needs. You can even integrate external applications and scripts to make your workflow even more streamlined.
- Portability: Once you create a bash script to perform a particular task, this bash script can work in any Linux distribution, provided the required dependencies are installed on that system. You can even use the bash scripts on other Unix-like systems like macOS and even on Windows via WSL.
- Integration: Bash scripts can be integrated with other tools like databases, APIs, or even with other bash scripts that help the users utilize the maximum potential of third-party tools for complex tasks.
- Easy to write: Bash scripts are very easy to write, thanks to easy syntax which Linux users are already familiar with. You do not need any special tools or software to write bash scripts which allows rapid prototyping of ideas and new tools.
- Low learning curve: Bash scripting has a relatively low learning curve, making it an ideal choice for both beginners and experienced users.
Basic Bash Script Components in Linux
If you look around, almost every bash script is composed of some common yet fundamental components that act as building blocks. These blocks add functionality structure to the entire script:
1. Shebang (#!)
Every bash script starts with a “Shebang line,” which specifies the path for the Linux interpreter that will be used. It is written as:
#!/bin/bash
2. Comments in Bash Script
Even though comments are simply ignored by the interpreter when the bash script is executed. They are simply used to provide some extra information about the code for its users. It also improves the overall readability of the code. To write a comment in a bash script, simply add an octothorpe (#) at the beginning of the line.
For example:
# This is a comment line
3. Variables
Variables in bash scripts help in storing different types of data temporarily in the memory. To assign a variable with a value in a bash script, use this syntax:
<variable_name>=<value_to_store>
For example, to store “John” inside the variable “name”:
name="John"
To access the value stored inside the “name” variable, simply add a “$” symbol in front:
echo "Hello, $name"
Here, the value stored inside the “name” variable gets plugged into the echo statement which then prints the final output as:
Hello, John
4. echo Command
The echo command is a simple yet useful command which is used to print values as output. The syntax to print using the echo command is:
echo <value_to_print>
5. If-Else in Bash Script
Sometimes you may need to take situational decisions depending on different conditions. In bash, you can take different decisions with if
, then
and else
commands with the following syntax:
if [ condition ]
then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
Here are the different character sets that you can use in bash for conditional scripts:
Character Set | Description |
---|---|
&& | Logical AND; Used to compare two conditions and returns true only if both conditions are satisfied |
-eq | Equals to; Used to check if two values are equal or not |
-ne | Not equals to; Checks if one value is not equal to another value |
-lt | Less than; Used to check if the first value is less than the second value |
-gt | Greater than; Check if the first value is greater than the second value |
-le | Less Than or Equals to; Checks if the first value is either less than or equal to the second value |
-ge | Greater Than or Equals to; Checks if the first value is either greater than or equal to the second value |
For example, to check if two values in a variable are equal or not:
if [ $a -eq $b ]
then
echo "Equal"
else
echo "Not Equal"
fi
6. Loops
Loops are used when you need to repeat a set of statements or commands multiple times. In bash scripting, you can use either a “For Loop” or a “While Loop” for your looping needs.
For Loop
A “For Loop” is a kind of looping structure that is used when you know exactly how many times you need to repeat a group of statements or use it to go through a list of things. The syntax to use the for loop in a Linux bash script is –
for <loop_variable> in <sequence>
do
# commands to be executed for each item in the <sequence>
done
Here’s a breakdown of the above syntax:
- <loop_variable> refers to the temporary variable which will hold each item of the <sequence> during each iteration. You can choose any name for the <loop_variable>.
- <sequence> contains the items over which the for loop will iterate. You can specify a <sequence> as follows:
- Range of numbers: It is a sequence of numbers that are equally spaced. You can generate a range using the following syntax: “{<start_number>
.. <end_number>}”. - List of items: You can specify an explicit list of items separated by spaces like
item1 item2 item3
- command output: Even the output from a command can be used as a <sequence> with the syntax as
$(<command>)
- Range of numbers: It is a sequence of numbers that are equally spaced. You can generate a range using the following syntax: “{<start_number>
- do signifies the start of the loop body containing the statements that you need to repeatedly execute.
- done demarcates the end of the loop.
For example, to print all integers between 1 and 5:
for i in {1..5}
do
echo "Number: $i"
done
While Loop
In general, while loops are used when you don’t know exactly how many times you need to repeat a particular set of statements. They will continue to repeat the statements until a particular set condition is met. The syntax to use the while loop is –
while [ <test_condition> ]
do
# commands to be executed while the condition is true
done
In the above syntax:
- <test_condition> refers to the condition which is tested before every iteration to check if it is satisfied or not. Once the condition is no longer satisfied, the loop will terminate without entering the loop body.
- do signifies the start of the loop body containing the statements that you need to repeatedly execute.
- done demarcates the end of the loop.
For example, to print all integers between 1 and 5:
i=1
while [ $i -le 5 ]
do
echo "$i"
i=$((i + 1))
done
How to Write a Bash Script in Linux
Now that you know about the basic building blocks of bash scripting in Linux, we can now move on to how to write a bash script for your Linux system.
Step 1: Create a New File
You can create a new file with any of the top text editors available for Linux. Make sure to give the extension to the script file as .sh. For example, to create the test named “test.sh” with the nano text editor.
nano test.sh
Step 2: Define the Shebang Line
A shebang line defines the path to the interpreter which you want to use for executing the bash script. It is the first line of every bash script and is written commonly as:
#!/bin/bash
OR
#!/bin/sh
If you need to use any other interpreter for your script, use this syntax:
#!/usr/bin/env <interpreter>
Note: It is not compulsory to add comments to every bash script you write, but they can improve the overall readability of the code. Use the octothorpe symbol to add comments to your script. Once the file gets executed, all comments get ignored by the interpreter.
Step 3: Add the Commands and Code
Now you can start adding the necessary commands and instructions with the help of bash components, all combined in a logical manner. Let’s say you need to automate the process of moving all the .txt files in a directory to a separate directory. Use this bash script to move all .txt files into a separate directory in your Linux system:
#!/bin/bash
# Create a directory to store text files
mkdir -p TextFiles
# Move all .txt files to the TextFiles directory
for file in *.txt
do
if [ -f "$file" ]; then
mv "$file" TextFiles/
echo "Moved: $file to TextFiles/"
fi
done
echo "File organization complete!"
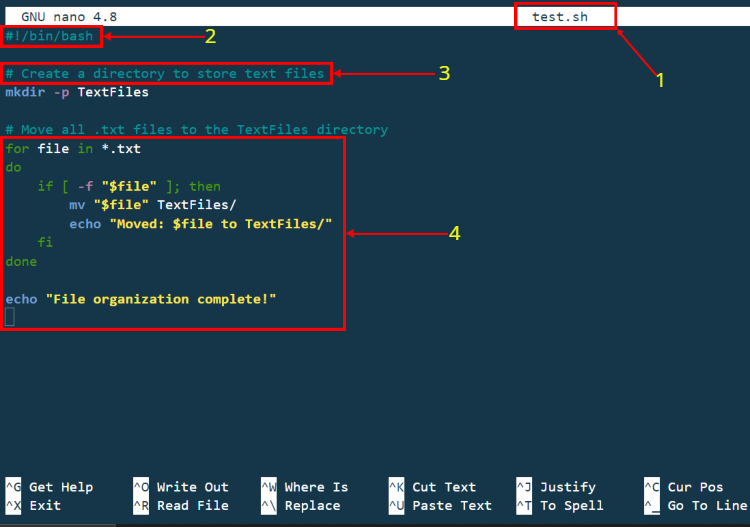
Seems complicated? Let’s break it down into parts:
#!/bin/bash
is the shebang line and specifies that the script is to be executed with the bash interpreter.# Create a directory to store text files
and# Move all .txt files to the TextFiles directory
are comments.mkdir -p TextFiles
creates a new directory named “TextFile” if does not already exists in the current directory.for file in *.txt
iterates over all .txt files with a for loopdo
signifies the start of the loopif [ -f "$file" ]; then
checks if the current item is a regular file or not- If the above condition is satisfied, then the current file is moved to the TextFiles directory with
mv "$file" TextFiles/
echo "Moved: $file to TextFiles/"
displays a message on the console indicating the file has been moved to the TextFiles directory
- If the above condition is satisfied, then the current file is moved to the TextFiles directory with
fi
indicates that the if block has finished executing for the current iteration.done
indicates that the for loop block has finished executing.- Once all txt files are moved, the final message is displayed on the console using
echo "File organization complete!"
Step 4: Save the Script
Once you have finished working on the bash script, save the file and exit the editor.
Step 5: Make the File Executable
Generally, all script files are not executable by all types of users in Linux. To make the bash script executable, use the following command:
chmod +x <filename>
For example, to make the file test.sh as executable:
chmod +x test.sh
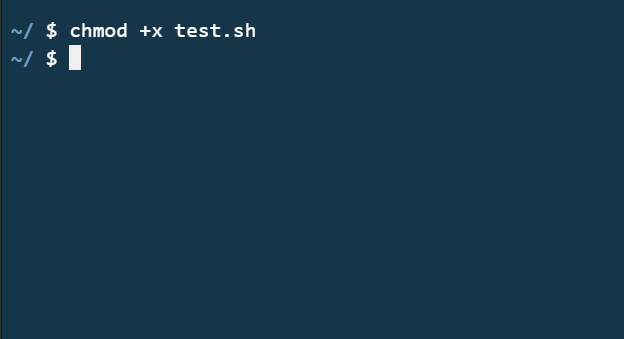
Step 6: Execute the File
Once you have made the bash script as executable, run the file with the following syntax:
./<filename>
To run the test.sh file:
./test.sh
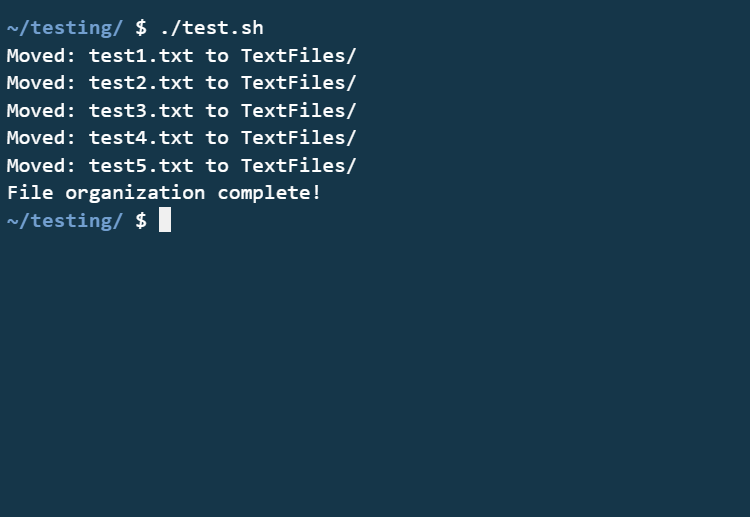
