Bash Scripts are essential for easing the lives of sys admins, developers, and Linux users in general. And an integral part of bash scripting is conditional statements, i.e. if, else if, and if else (elif) statements. These conditional statements enable Linux users to make decisions based on different conditions used inside the bash script, thus, providing much-needed flexibility and efficiency. That said let’s look at how to use If, Elif, and If Else bash statements with examples.
How to Use If Statement in Bash Script
The most common and fundamental conditional statement used to take decisions in bash script is the “if” statement. This is mainly used when you need to check if a certain condition is satisfied or not. The syntax to use the if statement in bash is:
if [[ <condition> ]]
then
<statement>
fi
In the above syntax, the <statement> gets executed only if the <condition> is satisfied. For example, if you need to print a message on the console after checking if the user input value is less than 10:
#!/bin/bash
echo -n "Enter a number: "
read num
if [[ $num -lt 20 ]]
then
echo "The value is less than 20."
fi
When the above script is executed, the user input value is checked whether it is less than 20 or not. If it is less than 20, then “The value is less than 20.” gets printed as output.
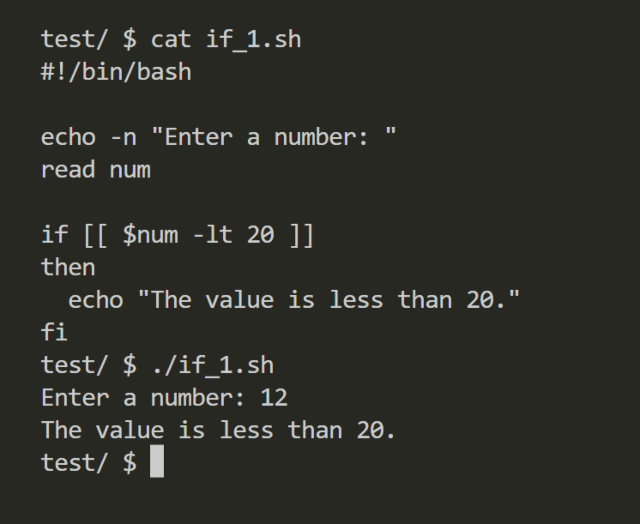
How to Use If Else Statement in Bash Script
So with the if statement, you can perform a certain operation as long as a condition is satisfied. But sometimes you may need to perform an operation if the condition is not satisfied. For this, you can use an “else” conditional statement in combination with the if statement to add more flexibility to your bash script for your Linux distro. The syntax to use the else statement is:
if [[ <conditon> ]]
then
<statement_to_execute_if_condition_is_true>
else
<statement_to_execute_if_condition_is_false>
fi
For example, to check if the user is eligible to vote or not:
#!/bin/bash
echo "Please enter your age:"
read age
if [ "$age" -ge 18 ]; then
echo "Congratulations! You are eligible to vote."
else
echo "Sorry, you are not eligible to vote yet."
fi
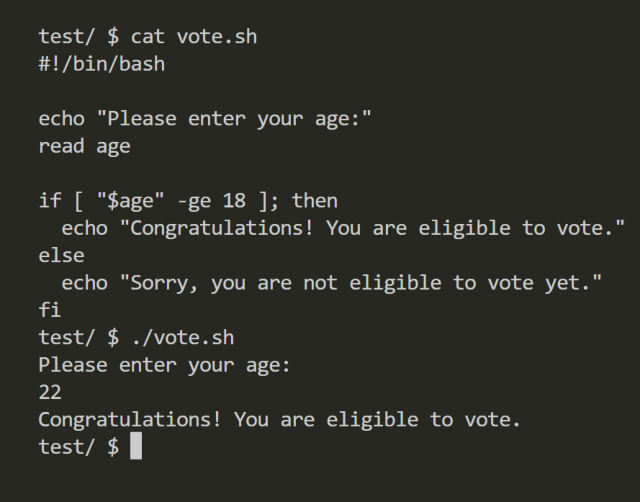
How to Use Else If (Elif) in Bash Script
While the if-else conditional construct is good for checking one condition and executing a task accordingly, what if you need to test multiple conditions? For such a situation, “else if” or “elif” statement comes into play. With elif, you can test multiple conditions and modify your bash script accordingly. The syntax to use the elif statement in bash is:
if [ condition_1 ]
then
<statements_to_follow_if_condition_1_is_true>
elif [ condition_2 ]
then
<statements_to_follow_if_condition_2_is_true>
else
<statements_to_follow_if_all_conditions_are_false>
fi
For example:
#!/bin/bash
echo -n "Enter a fruit name: "
read fruit
if [ "$fruit" = "apple" ]
then
echo "It's an apple."
elif [ "$fruit" = "banana" ]
then
echo "It's a banana."
else
echo "It's neither an apple nor a banana."
fi
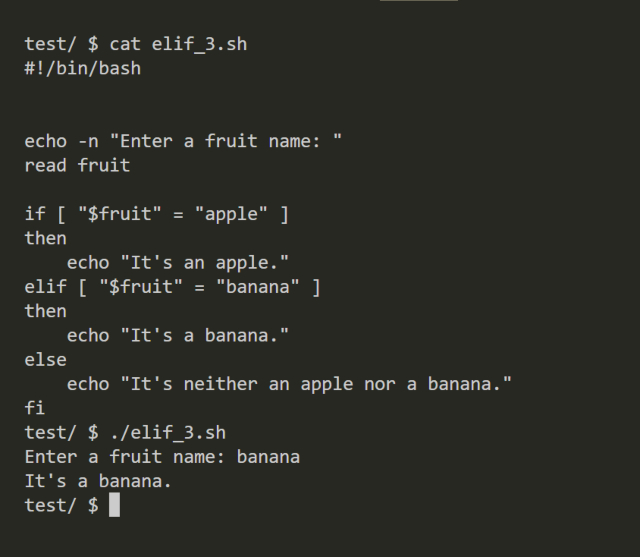