Suppose you are working on a complex bash script to automate a series of tasks on your Linux system. You notice that as it grows, there are certain parts of the script where the same code blocks are repeated multiple times. For this, you can wrap the repeating piece inside a block known as a ‘function’ and reuse it, making the overall code more streamlined and organized. In this article, we will discuss what is a function in bash and how to use it for automating Linux tasks.
What are Bash Functions in Linux?
Bash functions are reusable code blocks in a bash script that can be grouped together and named to avoid writing the same code repeatedly. Much like other programming languages, you get the option to use functions in your bash scripts. Functions help you organize your entire script into smaller modules, making your code more readable, easy to debug, and reusable. In other words, functions are like a script within a script.
How to Define Functions in Bash?
Now that you have a basic idea of what are bash functions, let’s see how to define them to make our Linux workflow much easier. The basic syntax to define bash functions are:
<function_name>(){
<statements_to_execute>
}
You can even use the single-line version of the above syntax:
<function_name>() { <statements_to_execute>; }
Some points to remember about defining bash functions include:
- Every statement and command which are written inside the {} is known as the function body. For the function name, you can use anything except a keyword which are specific words reserved for different commands.
- Unlike conventional bash script, functions do not get executed unless you call them. To execute a function, you need to use the function name.
- When using the single-line version, every command you write must be separated with a semi-colon ‘;’.
- All variables declared inside a function can only be accessed inside the body of the function.
Let’s see a basic example based on the above syntax. Start by creating a new file in Linux text editors and write this code inside it:
#!/bin/bash
hello_world () {
echo 'hello, world'
}
hello_world
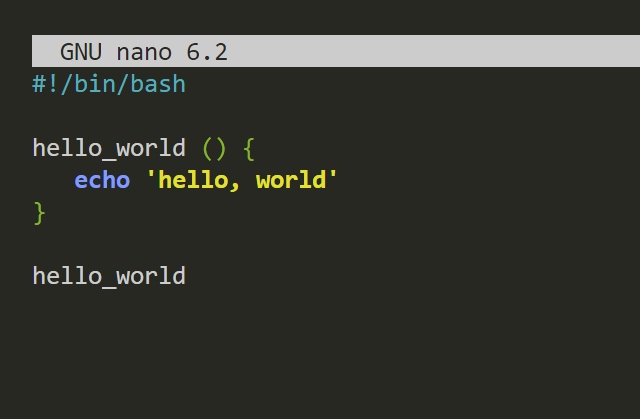
It is recommended to save the file with the same name as the function name. To invoke the file, simply write the function name inside the shell prompt and hit enter.
./hello_world
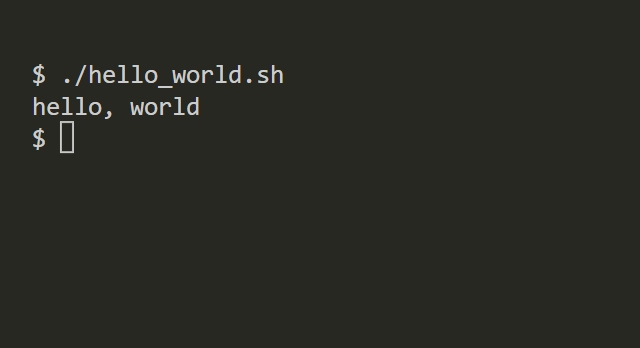
How to Pass Arguments to Bash Functions
Sometimes you may need to work with some custom values or work with the user input values with the functions you’ve created. To pass arguments to the bash function, simply mention them after the function name after invoking the function as Linux shell variables. As for using the arguments inside the function, place them with $<argument_position>
, like $1
, $2
below and so on. For example:
#!/bin/bash
greeting () {
echo "Hello $1"
}
greeting "Beebom"
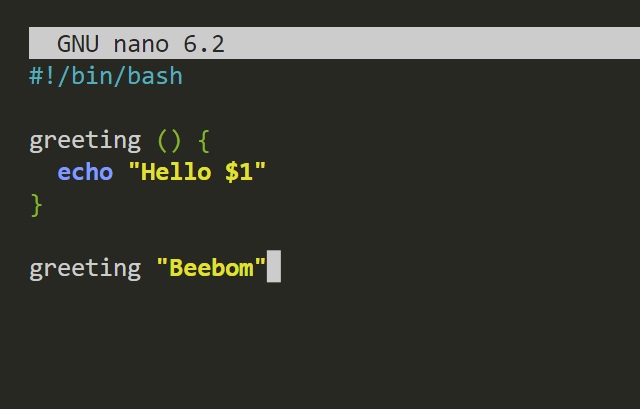
To invoke, use the following command:
./greeting.sh
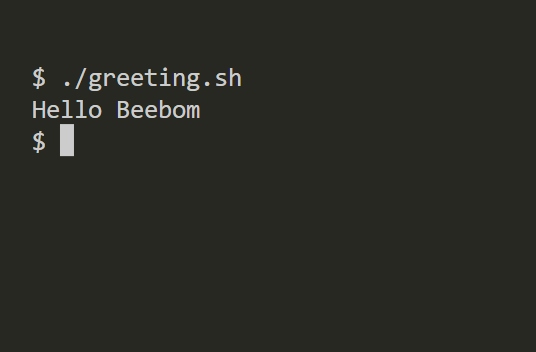
How to Return Value from Bash Functions
Unlike other programming languages, in bash, you cannot return values from the function. You can only send the return status from the bash function to the caller with any number ranging from 0 to 255, where 0 represents success and any other number as a Linux system error/failure. For example, copy this code in the nano editor in Linux.
#!/bin/bash
greeting () {
echo "Hello $1"
return 10
}
greeting "Beebom"
echo The return value is $?
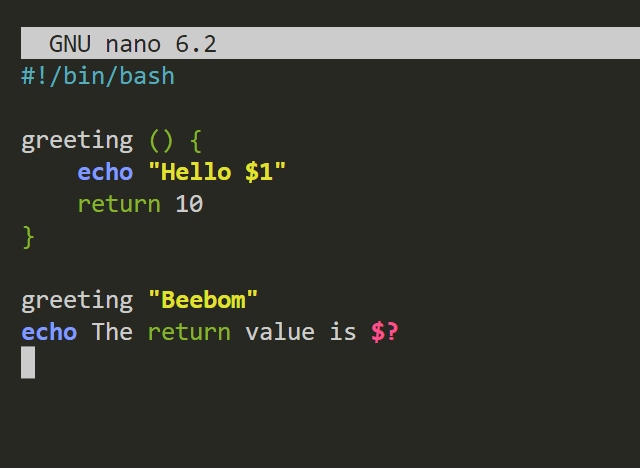
When you call the above script, you will get a similar output along with the value 10, indicating a failure or error has occurred.
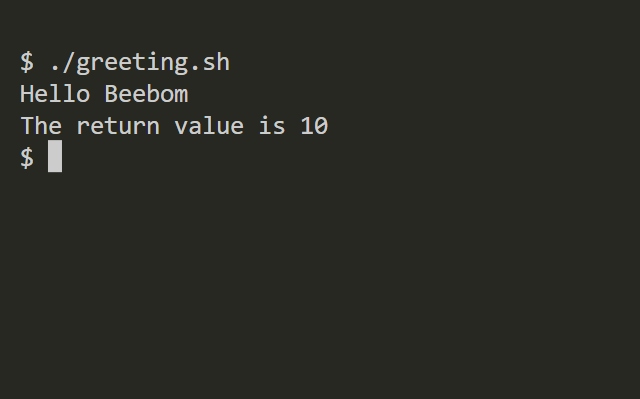
Variable Scope in Bash Functions
The scope of a variable means code parts where the variables can be used in bash. The scope can be divided into mainly two parts – global and local scope. With the global scope, the variable can be used everywhere including directly in the shell prompt. Local scope means the variable cannot be used outside the block where it is declared.
Every variable you declare inside a bash function with the “local” keyword will fall under the local scope of the function body and cannot be used outside of the function body. Similarly, global variables can be accessed from anywhere in the bash script without any restrictions. The following example will make it clear:
#!/bin/bash
global_var="I am global"
function display_global() {
echo "Inside function: $global_var"
local local_var="I am local"
echo "Inside function: $local_var"
}
display_global
echo "Outside function: $global_var"
echo "Outside function: $local_var"
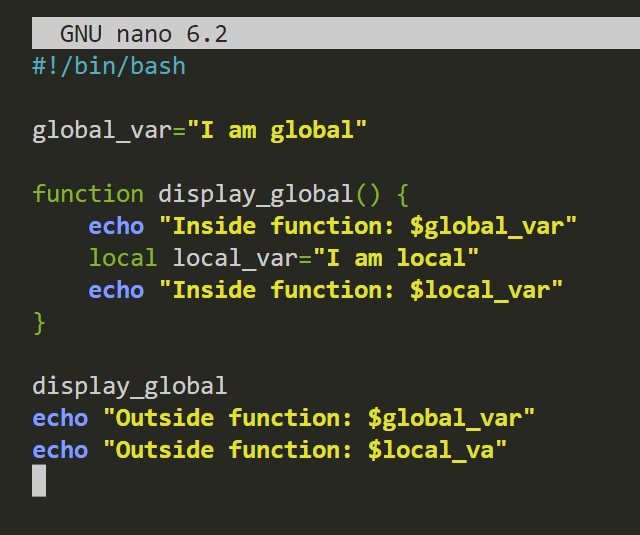
After executing the above script, you will see the following output:
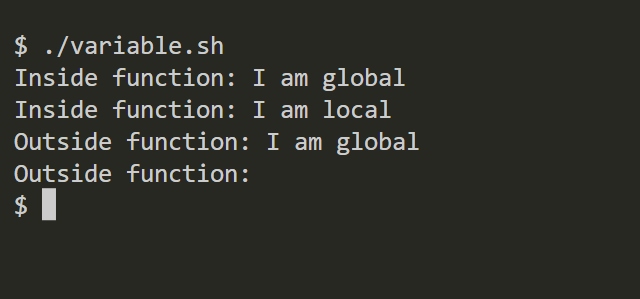
Best Practices to Use Functions in Bash
Bash functions are powerful tools that can enhance the structure, maintainability, and reusability of bash scripts in Linux. Follow these best practices to use functions in bash that can vastly improve our workflow:
- Keep functions short and focused: Try to keep the functions you write short, concise, and focused to perform a single task. This will improve the overall readability and maintainability of the bash script.
- Use descriptive names: Use a short but descriptive name that reflects the purpose of the function as the function name. Clear and meaningful names make the code more self-explanatory, allowing you as well as other developers to maintain and build on the code.
- Add comments: Not just for bash scripts, but for all different types of programming languages you work on, use comments describing which part of the code is doing what.
- Reuse functions: Reuse functions wherever possible to avoid rewriting the same code over again.
- Avoid function name clashes: Avoid using functions with the same name as the built-in commands and other command-line tools.